npm ERR! Missing script: “start” [SOLVED]
Node.js is an excellent tool for creating RESTful APIs. You were probably working with Node.js and a package manager like npm or yarn when you ran into an error like npm ERR! Missing script: "start"
or a variant like npm ERR! Missing script: "dev"
, npm ERR! Missing script: "test"
, or npm ERR! Missing script: "build"
.
Why do these kinds of errors happen? The truth is that they can occur for a variety of reasons.
Table of Contents
5 common causes: npm ERR! Missing script: “start”
Here is a list of the most common causes of the npm ERR! Missing script: “start” error.
- You opened your code editor (IDE) in a folder that does not contain your project’s
package.json
file - You opened your command line program in a folder that does not contain your project’s
package.json
file - You don’t have a
package.json
file for your project - You don’t have a
start
command in the scripts section of yourpackage.json
file - Having more than one
scripts
object inpackage.json
Now, how to fix this error?
How to fix npm ERR! Missing script: “start”?
Were you trying to start a Node.js app with the npm start
command? If this is the case, be sure to follow the steps below.
- If the error appears in the code editor (for example, an IDE like Visual Studio Code), you are most likely using an Integrated Terminal. Make sure that you opened the code editor in your project directory and that in the Integrated Terminal you are also in your project directory. Inside this folder, you should find the
package.json
file - If the error appears in a command line program, such as Powershell, Terminal, or iTerm2, make sure you are in the correct folder. Inside this folder you should find the
package.json
file
If you try to run the npm start
command from a different directory, npm
will not find the package.json
file and will throw the error Missing script: "start"
.
- If you already verified you are in the correct directory, is there a
package.json
file inside this folder? If you don’t have apackage.json
, you can create one in your command line program by running the following command:npm init -y
- If you already are in the appropriate folder, open the
package.json
file, and check thescripts
section and make sure it has astart
command. Yourpackage.json
file should have a section similar to the code block below. Pay special attention to howscripts
has astart
property.
{
"name": "demo-node-ts",
"version": "1.0.0",
"scripts": {
"start": "node index.js"
},
"devDependencies": {
"jest": "29.5.0"
}
}
- Another common reason is having more than one “scripts” object in
package.json
. This causes an error because the second “scripts” object overrides the first. If you have twoscripts
objects, you may only have thestart
command on the firstscripts
object. Confirm that you only have onescripts
object.
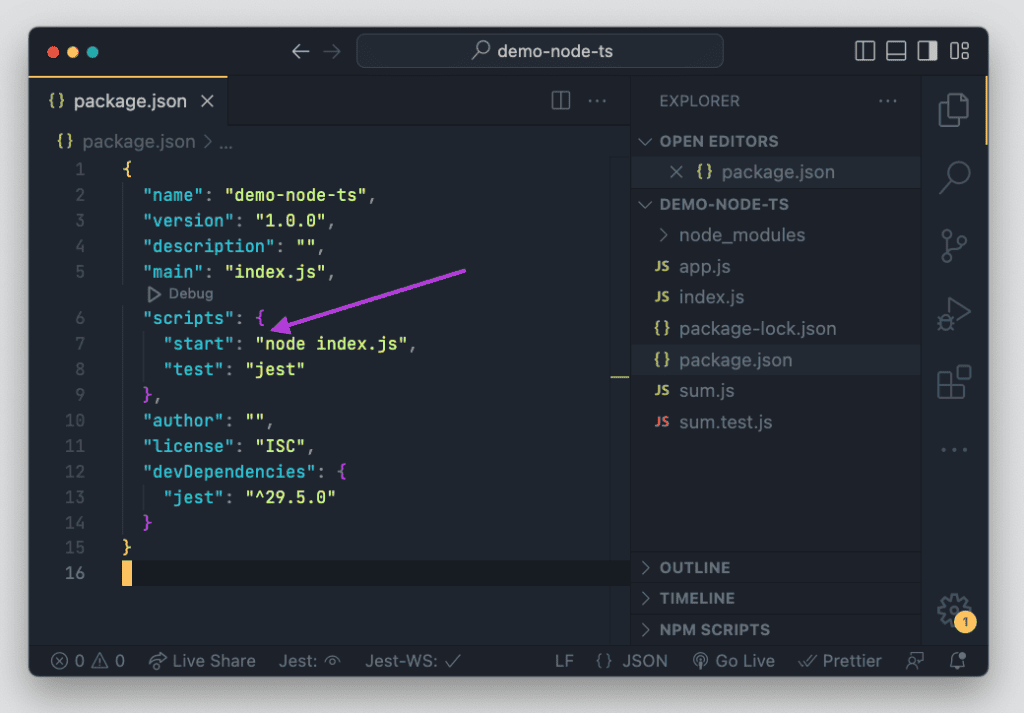
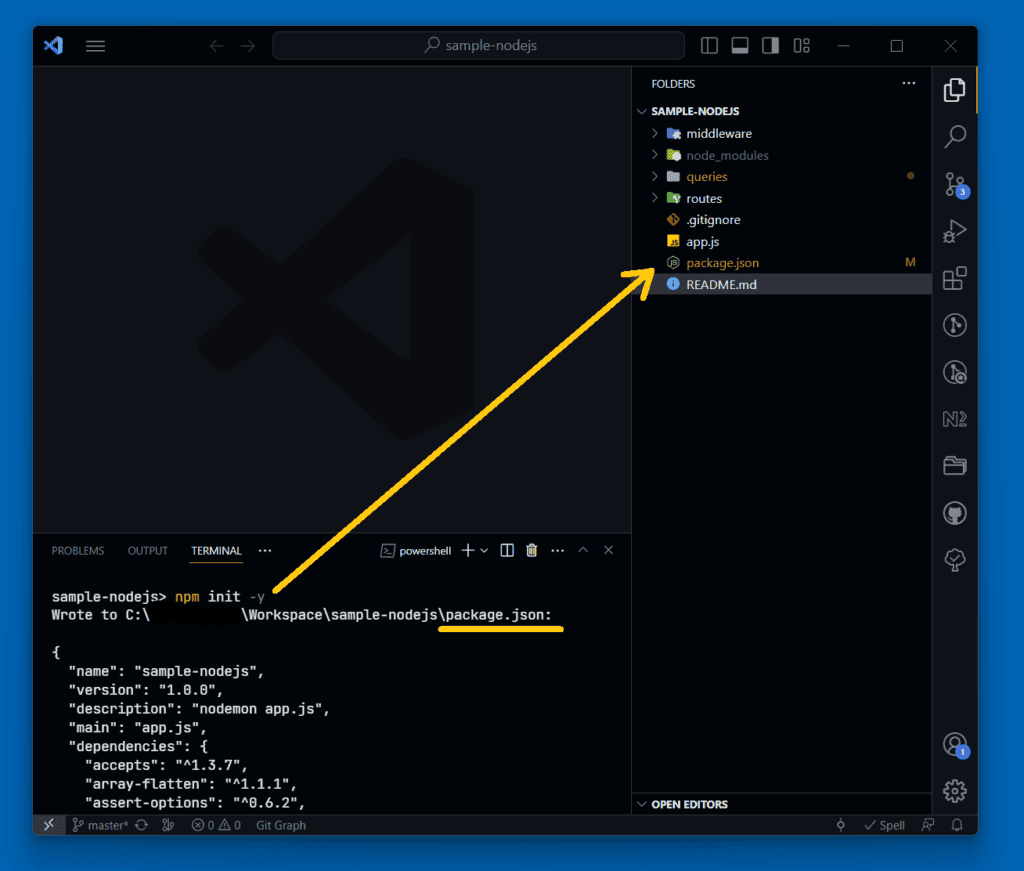
npm init
initializes a new Node.js project and generates a package.json
file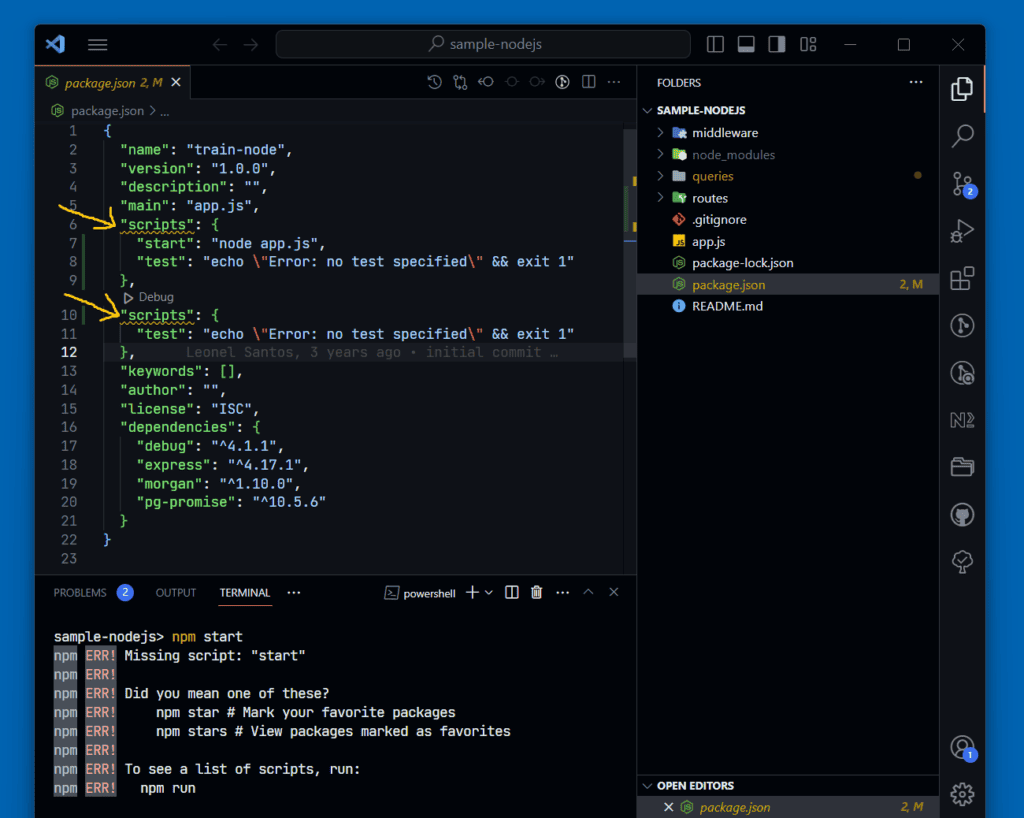
package.json
file with more than one scripts
object. The second cancels the first.Typically, each Node.js project has its own package.json
file. package.json
is where metadata about the project is specified, such as the project name, version, its dependencies, and scripts that point to other files to run.
npm ERR! Missing script: “dev” and similar errors
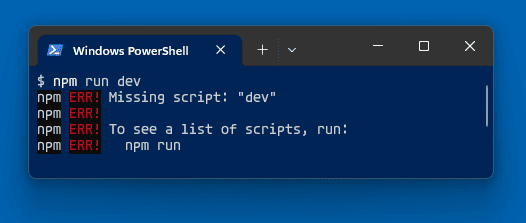
npm ERR! Missing script: "dev"
in Windows PowerShellSometimes you may run into similar errors such as the following:
- npm ERR! Missing script: “dev”
- npm ERR! Missing script: “build”
- npm ERR! Missing script: “test”
- npm ERR! Missing script: “lint”
- npm ERR! Missing script: “watch”
The root cause of these failures is the same as the ones for npm ERR! Missing script: "start"
. Take a look at what has already been said in this article about the causes of that error and its possible solutions.