How to use reduce in Javascript? [5 EXAMPLES]
The name of the function reduce()
confused me, I assumed it subtracted elements from an array, which I now know it’s wrong. Are you also confused by the reduce
function? Now that I understand how it works, I’ve found it to be a very useful feature.
In this post, I will explain how to use Array’s reduce
function in Javascript and show you practical ways to use it.
Table of Contents
How does reduce work in Javascript?
The reduce
method performs a cumulative action on an array. It does so by executing a function on each element of the array, returning a single value as a result.
The reduce
function can receive 2 arguments:
- callback function: this callback function, in turn, can receive 4 arguments
- previous value, also called accumulator
- current value
- current index
- array
- initial value
reduce’s syntax (simplified)
Since what I want to achieve is that you understand the reduce
function, here is a simplified syntax, showing only the most common arguments.
array.reduce(callback(previousValue, currentValue), initialValue)
callback function: function to execute on each element of the array. The value it returns becomes the previousValue
on the next callback invocation.
- previousValue: The value returned by the callback on the previous invocation. The first time the callback is invoked, it is equal to initialValue.
- currentValue: the value of the current element
initialValue: is the value to use as previousValue
in the first call to the callback function.
An EASY example to learn how to use reduce
One of the easiest ways to understand how reduce
works is to use it to add numbers from a list.
const array = [2, 8, 4, 1];
const sum = array.reduce((previousValue, currentValue) => {
return previousValue + currentValue;
}, 0);
console.log(sum); // ➡️ 15
The callback function is executed 4 times:
- previousValue: 0, currentValue: 2, returns 2
- previousValue: 2, currentValue: 8, returns 10
- previousValue: 10, currentValue: 4, returns 14
- previousValue: 14, currentValue: 1, returns 15
Can you visualize the sequence of calculations in each callback execution?
0 + 2 = 2
2 + 8 = 10
10 + 4 = 14
14 + 1 = 15
Aside from using reduce
to calculate the sum of an array of numbers, there are many other practical ways to use it.
How to use reduce with an array of objects?
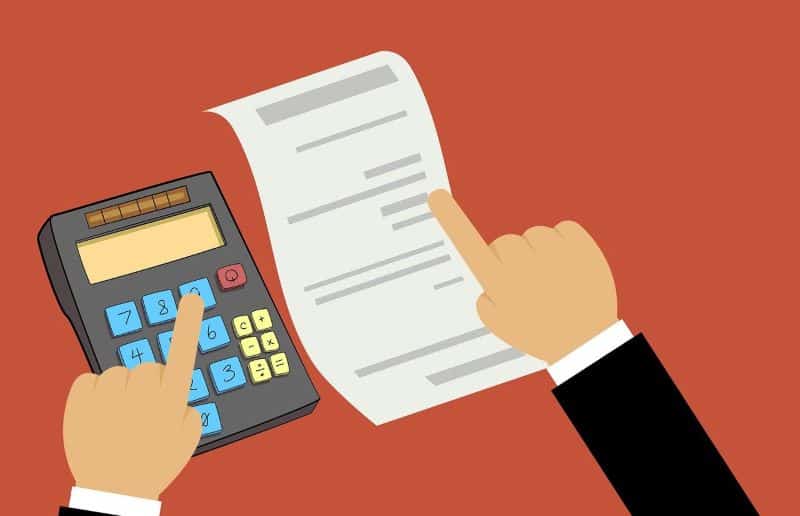
For instance, you can use reduce
to calculate an invoice’s total.
const invoice = {
customer: 'Company ABC',
date: '2023-09-08',
products: [
{ name: 'Coffee beans bag', quantity: 2, price: 250, },
{ name: 'Mechanical keyboard', quantity: 1, price: 100, },
{ name: 'Glass cup', quantity: 1, price: 100, },
],
};
Note that you also have to consider the quantity to calculate the invoice’s total.
const total = invoice.products.reduce((previous, current) => {
return previous + (current.quantity * current.price)
}, 0);
// total is 700
You can access the code in action on StackBlitz: calculate an invoice’s total.
Use reduce
to remove duplicate values from an array
Suppose you have this array:
const arr = [
'A', 'B', 'C', 'A',
'B', 'B', 'D', 'C',
];
How would you get rid of values that appear more than once and keep the unique array values? In addition to using reduce
, we will be using another Array method, includes
. Basically, this last method determines if an array includes a certain value or not, and returns true
or false
accordingly.
const uniqueValues = arr.reduce((unique, letter) => {
if (unique.includes(letter)) {
return unique;
}
return [...unique, letter];
}, []);
Here’s a concise version using the ternary operator.
const uniqueValues = arr.reduce((unique, letter) =>
(unique.includes(letter) ? unique : [...unique, letter]),
[]);
How do you count the number of times an element appears in an array?
To count the occurrences of array elements by using reduce()
we can do something like the following:
const fruits = [
'Banana',
'Apple',
'Orangle',
'Apple',
'Strawberry',
'Banana'
];
const counts = fruits.reduce((acc, curr) => {
return {
...acc, [curr]: (acc[curr] || 0) + 1,
};
}, {});
// Outputs the following object:
// { "Banana": 2, "Apple": 2, "Orange": 1, "Strawberry": 1 }
Note that we are using the spread syntax and the OR operator ||. You can see this same example on StackBlitz: counting the number of times a value appears in an array.
Find the maximum and minimum value in an array
You can easily get the maximum and minimum values of an array with just a few lines of code using reduce
.
To illustrate, let’s say you have an array of teams with their respective scores.
const teams = [
{ name: 'Tigres', points: 74 },
{ name: 'Los Bots', points: 55 },
{ name: 'Rockets', points: 80 },
{ name: 'Visible Wifi', points: 91 },
{ name: 'The Coffee Drinkers', points: 63 },
];
How would you find the maximum score?
const max = teams.reduce((previousTeam, team) => {
if (previousTeam === null || team.points > previousTeam) {
return team.points;
}
return previousTeam;
}, null);
The variable max
would have the value 91
.
At each iteration, we check if the number of points is greater than the previous value, or if the previous value is null
. If so, we update the accumulator with the higher score.
In other words, we simply return the highest score at each iteration.
Now, how would you find the minimum score? There is only one small change to make, try to identify it.
const min = teams.reduce((previousTeam, team) => {
if (previousTeam === null || team.points < previousTeam) {
return team.points;
}
return previousTeam;
}, null);
The variable min
would end up pointing to the numeric value 55
.
The only difference between finding the minimum or maximum value lies in the operator: from greater than (>
) to less than (<
).
You can find the full example on StackBlitz: Finding max and min values in an array using reduce.