How to parse command line arguments in Node.js?
It’s quite common to run applications from the command line using arguments. Usually we don’t want the app to do the same thing every time it runs, we want to configure its behavior with arguments and/or flags.
In this tutorial, we’ll explore how we can handle command line arguments in Node.js.
Table of Contents
What is argv in Node.js?
In Node.js all command line arguments received by the shell are passed to the process in an array called argv
, which is short for “argument values”.
How to use argv?
For each running process, Node.js exposes an array with the values of the command line arguments in the form of process.argv
If you already have Node.js installed, try the following, it’s easy.
Create a file called main.js
and add this line:
// main.js
console.log(process.argv);
Save the file and try the following in your shell:
$ node main.js one two=three four
[ '/Users/leo/.nvm/versions/node/v16.17.1/bin/node',
'/Users/leo/workspace/main.js',
'one',
'two=three',
'four' ]
There you have it: an array that contains the arguments you passed.
Take a look at the array elements:
process.argv[0]
contains the absolute path to the executable that started the Node.js processprocess.argv[1]
contains the path to the running JavaScript file, in this casemain.js
- the elements that follow have the values passed by the command line
The path to the executable and the path to the running JS file will always be present, even if your program doesn’t take any arguments of its own, the interpreter and path to your script are still considered arguments to the shell you’re using.
In most cases, you won’t need the path to the executable or the path to the file. How to omit the first two elements of the array? Try the following in main.js
:
const args = process.argv.slice(2);
console.log('args: ', args);
The slice method extracts the elements starting with index 2 and up to the end of the array, then returns a copy of the array with the extracted values.
The previous code outputs the following:
$ node main.js one two=three four
args: [ 'one', 'two=three', 'four' ]
Important note regarding process.argv and data types
What happens if we pass numeric or boolean values on the command line? Try this on the command line:
$ node main.js 1 2 true false
It produces:
args: [ '1', '2', 'true', 'false' ]
Can you notice what happened? You may have expected that 1 and 2 would be numeric type values and true
and false
would be boolean type values. But what do we have? The array elements in process.argv
are string type values.
Keep in mind that process.argv
will always return an array with elements of type string. This is precisely what the official API reference documentation for Node.js states:
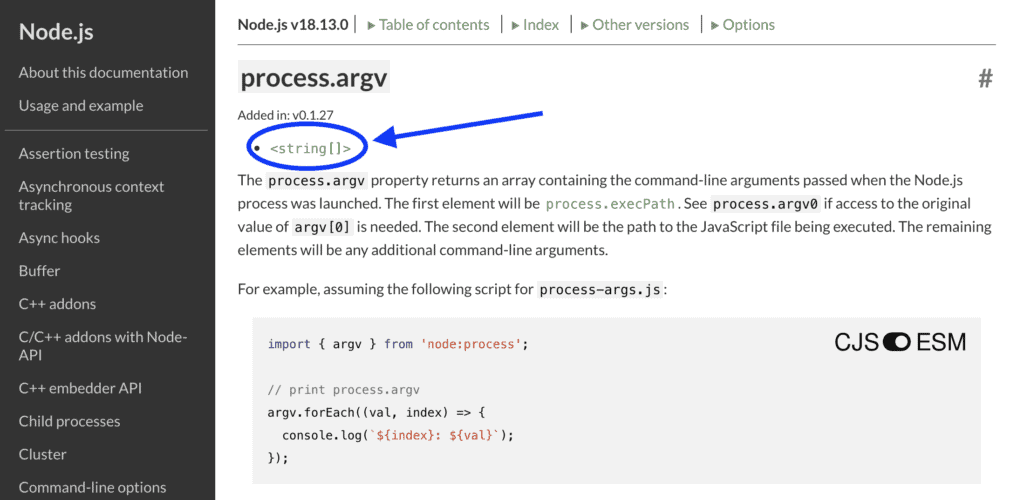
How to use yargs to simplify argument parsing?
Referencing command line arguments by array index is not very clear and can quickly become a nightmare when you start working with multiple of arguments and flags.
Imagine you created a server and it needs a lot of arguments to start. Now you have to deal with something like this:
myApp -h host -p port -r -v -b --quiet -x -o outfile
Some flags need to know what’s coming next, some don’t, and most CLIs allow users to specify arguments in any order they like 😱
Sounds like a fun array to analyze?
Fortunately, there are plenty of third-party modules that make all of this trivial, one of which is yargs
. It is available through npm.
For more information on yargs and the many other things it can do for your command line arguments, visit the official Yargs site.